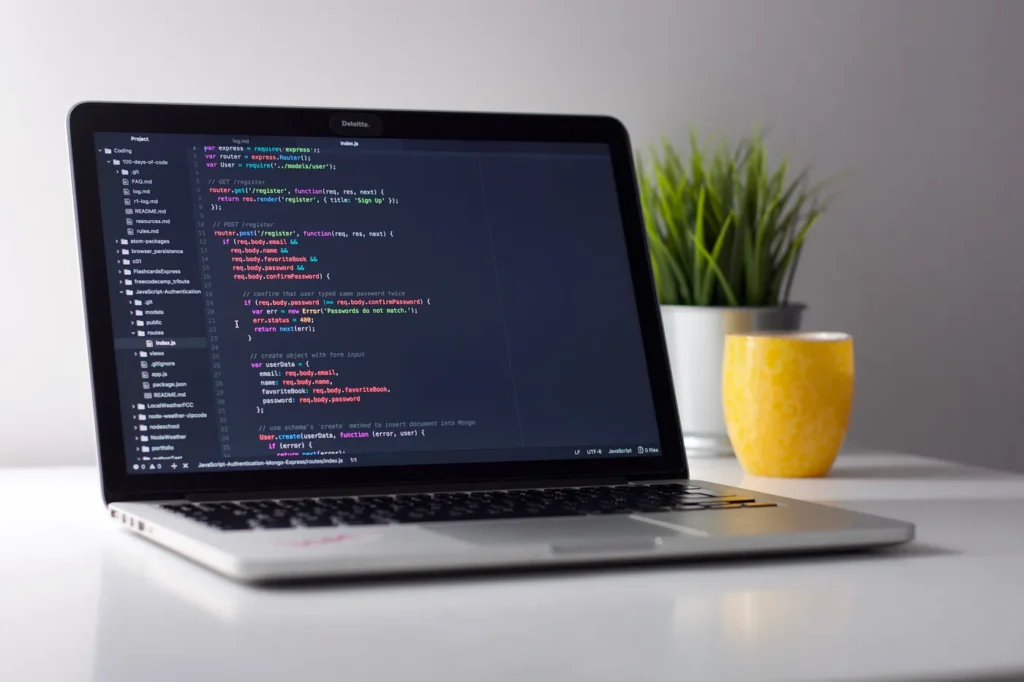
This is a follow up to progressively improving .net MVC with Vuejs to show how it can be done. The screen shot below shows how we can use a mix of razor templates and vuejs in the same page.
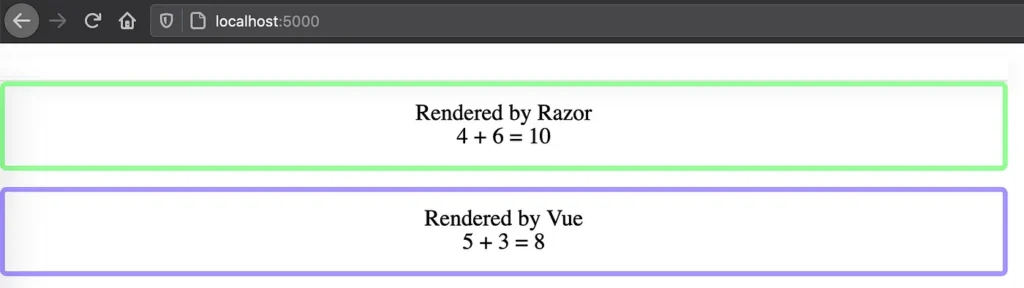
Above you can see two divs where 2 numbers are being added. One part is being rendered simply in the Razor template where as the other involves passing the 2 values to vuejs which adds them by using an async API call to an MVC controller. Lets look at the code.
The razor bit is pretty straight forward, for vuejs we are passing some contextual information using data tags. In this case we pass values to add and the base api url that our vue app can use to make the API call to add. The last two lines are static assets for our Vuejs App which are generated from our Node project. This bring up a few questions.
How to generate vue components for every page?
VueJs can be configured for a multi page setup. It allows us to tell Vue to generate JS for every component that we want to be able to render outside from vue. In this case lets say we have an Add page and want to generate JS for this page. To do this we can use the vue.conf.js which allows us to define those.
This will generate a JS file in the format of main.{sha}.js in the dist/js folder and the content will be src/pages/add/main.js file which we will discuss next.
How do we pass data from MVC to Vue?
The main file acts as the glue and reads the data tags of DOM element with id addContainer. It then gets all the required values using data tags an then passes them to the context of the Add component being rendered by VueJs. This allows us to communicate from .net MVC to VueJs. We restricted this to only basic contextual data.
How do we serve the generated js?
We just need a basic script which can parse the vue.config.js and output them to the relevant folders in MVC. For this example I have just put a simple script to send the files across. It builds the vue app and copies across the js assets before starting the MVC app.
What about the rest?
Getting those earlier issues out of the way allows our Vue code to just work as any other Vue app. Below is the code for the Vue component.
The component receives the values to add and the base API URL. It initially shows “Calculating…” on load. Once the component has been created it uses the created lifecycle to make and API call and update the template with calculated value from the server. This is how we can make API calls to the server. Lets Look at the controller method which adds the two numbers.
The controller has two methods. The Http GET returns the Razor view which in turn will also load the Vuejs app. The Http POST method accepts a payload which contains the values to add. It returns a json response which contains the sum.
Final thoughts
As you can see this way gives us the flexibility to use vuejs inside an existing MVC app. It allows for a mix of server rendered vs client rendered app. This also gives us all the benefits of using a newer js framework where required.
Here is the link to the code for you try out on your machine.