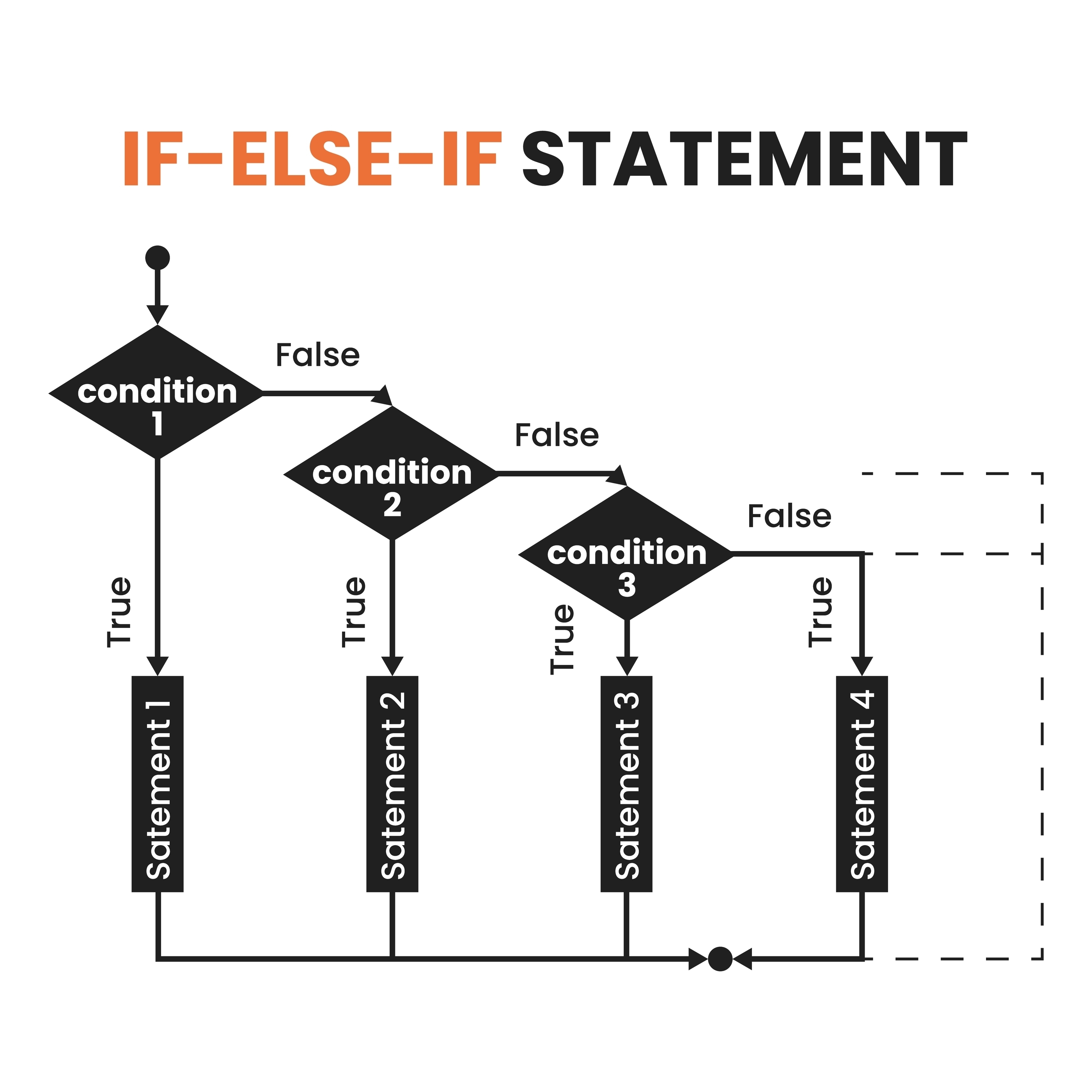
The Problem
Imagine you’re building a product that helps people post their content across multiple social media platforms, like Facebook, X (formerly Twitter), LinkedIn, and others. At first, you might think, “I’ll just write some code that checks which platform the user wants to post to and then handle each one separately using if-else or switch-case statements.”
For example:
If it’s Facebook, do this
If it’s LinkedIn, do that
If it’s X, do something else
This might work in the beginning. But as your business grows, new social media platforms will emerge. Platforms you’ve never heard of today may become the most popular ones tomorrow. Once a new one comes along, you will again go to your codebase and do the following:
Adding more if-else statements
Modifying existing code, which could unintentionally introduce bugs
Dealing with a complex web of logic that becomes difficult to understand and manage
It’s like adding rooms to a house without any proper planning, you can do it, but the house will soon become chaotic and hard to live in.
What if there was a smarter way?
This is exactly the kind of problem the strategy pattern solves. It allows you to create a system where adding support for a new platform is as simple as plugging in a new piece, without touching the rest of the system.
What options do we have
First, let’s explore the options available to us:
if-else
switch case
Though they may be easier options, there are a few problems with using them. They are as follows:
Scalability: The more conditions there are, the harder they are to read and maintain.
Violation of Open/Closed Principle: Adding new conditions requires modifying the existing code.
Tight Coupling: There is less flexibility because the selection reasoning is closely tied to the context.
Testing Difficulty: Complex conditions make the unit testing harder.
Readability: Long chains decrease readability, making code harder to modify or debug.
In this type of scenario, we can use the Strategy Pattern to overcome the limitations of if-else and switch-case structures.
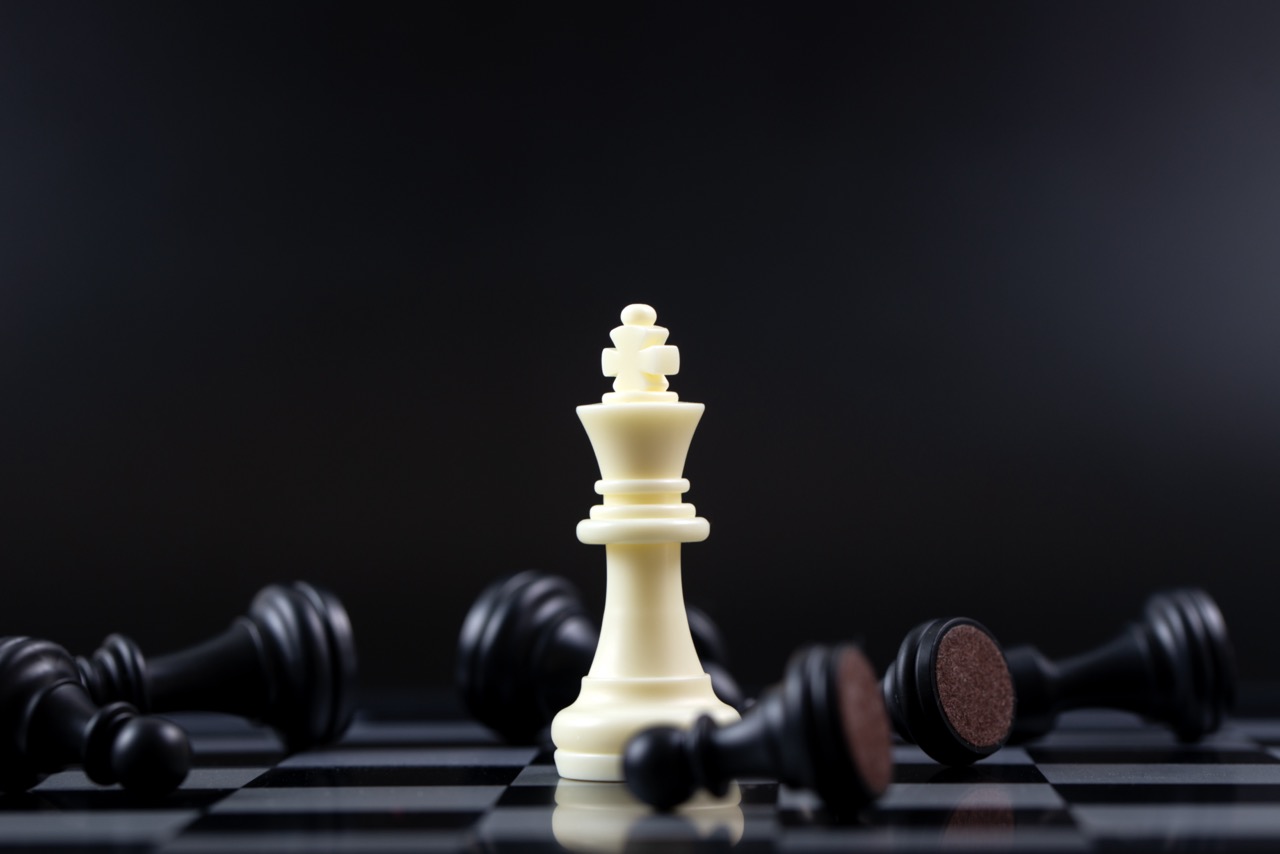
What is a Strategy Pattern?
The strategy pattern is a behavioural pattern, which deals with how objects behave and interact. The strategy pattern deals with using different implementations interchangeably according to scenarios. The code that is currently running decides what needs to be used, instead of things getting decided during compile-time, the code decides the logic during runtime.
Key Components in the Strategy Pattern
There are a few components in the strategy pattern. They are as follows:
Context
Strategy
Concrete Strategies
Let us look at what each one of them does.
Context: The context, as such, doesn’t hold any important implementation logic, rather it has reference to all the different implementations. It knows there are different implementations but not the actual implementation. During runtime, it delegates the correct implementation to be used. It helps in changing between different implementations.
Interface: The interface is the core of the strategy pattern. It acts as a contract saying what the different strategies must implement. It defines the common behaviour. All the implementations are the implementation of this interface.
Concrete Strategies: Several implementations are implementing the above discussed interface. These implementations have the logic for different implementations. These concrete strategies are the ones that are chosen during runtime based on certain conditions.
Implementing the Strategy Pattern
Now, let us look into implementing a strategy pattern for the problem of sharing to different social media platforms.
Step 1: Creating the Interface
// SharingStrategy Interface
interface SharingStrategy {
fun shareContent(content: String)
}
Step 2: Creating different concrete implementations
// 1. Facebook
class FacebookSharing : SharingStrategy {
override fun shareContent(content: String) {
println("Sharing on Facebook: $content")
}
}
// 2. Twitter
class TwitterSharing : SharingStrategy {
override fun shareContent(content: String) {
println("Tweeting: $content")
}
}
// 3. Instagram
class InstagramSharing : SharingStrategy {
override fun shareContent(content: String) {
println("Sharing on Instagram: $content")
}
}
// 4. LinkedIn
class LinkedInSharing : SharingStrategy {
override fun shareContent(content: String) {
println("Sharing on LinkedIn: $content")
}
}
Step 3: Creating the Context
class ContentShare {
private val strategies = mutableListOf<SharingStrategy>()
fun addStrategy(strategy: SharingStrategy) {
strategies.add(strategy)
}
fun shareContent(content: String) {
for (strategy in strategies) {
strategy.shareContent(content)
}
}
}
Step 4: Client code that calls
fun main() {
// Create a content-sharing instance
val contentShare = ContentShare()
// User selects strategies based on preferred platforms
contentShare.addStrategy(FacebookSharing())
contentShare.addStrategy(TwitterSharing())
contentShare.addStrategy(InstagramSharing())
contentShare.addStrategy(LinkedinSharing())
// Share content across selected platforms
// Shares to Facebook, Twitter, Instagram & Linkedin
contentShare.shareContent("Check out my new blog post!")
}
Conclusion
In this blog, we’ve looked at the Strategy Pattern, a robust design pattern that promotes flexibility and maintainability in software development. By encapsulating implementations into separate classes, the Strategy Pattern allows developers to choose and switch behaviours at runtime without modifying the core logic as well as adding new implementation doesn’t break the existing flow.
Thank you for reading! Happy coding!