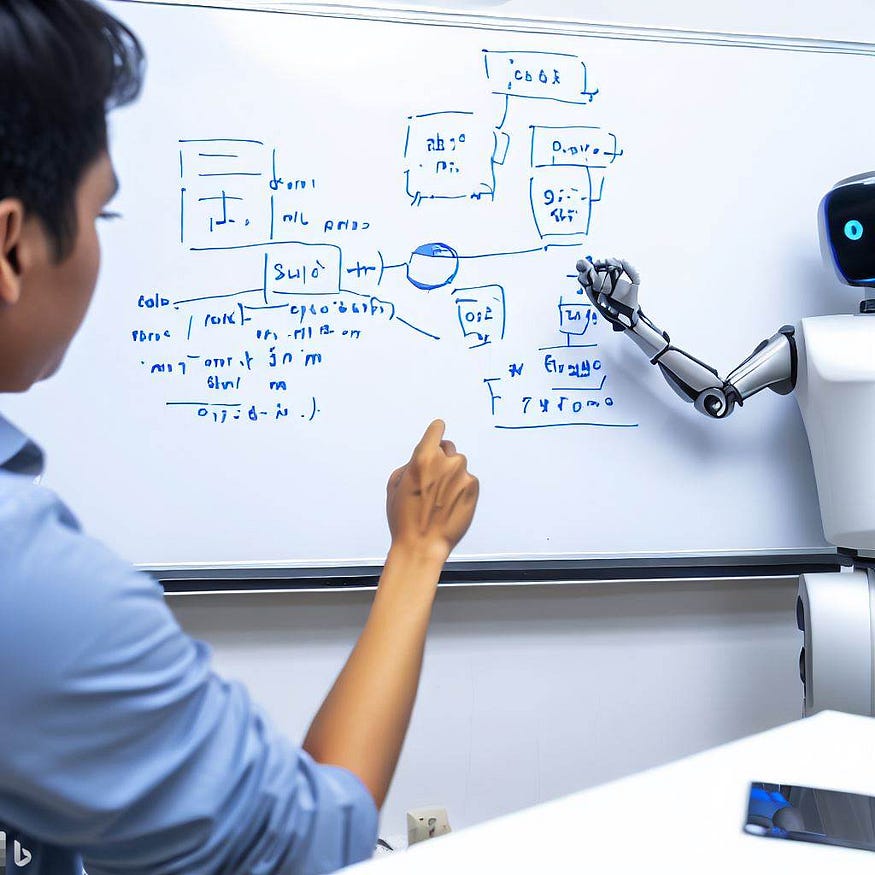
This article is part of a series where we explore how Generative AI can be utilized for the various stages in the software development lifecycle. You can read this post about the methods and tools used for experimentation.
Software architecture is essential when building software; it ensures that the developed software satisfies the business needs and sets up technology teams for success. This article explores how Generative AI, specifically GPT, can assist in software architecture.
Software Architecture is typically handled by engineers with different designations such as Software Architect, Principal Engineer, Staff Engineer, Tech Lead, or Senior Engineer, depending on the organisation and team structure. For this article, we will refer to these individuals collectively as “Software Architects”.
Key Responsibilities of a Software Architect
- Tech Stack Selection: Choosing the technology stack including the programming languages, frameworks, platform and databases.
- Decision Making: Making strategic technical decisions for design patterns, coding standards and deployment.
- System Design: Designing the overarching structure of the software system aligned with business goals.
- Cross-Functional Requirements: Integrating cross-functional requirements like security, scalability, performance and reliability into the software design.
- Risk Management: Identifying and mitigating potential risks in the system.
- Team Guidance: Guiding and mentoring the development team to ensure adherence to the defined architecture and coding standards.
- Stakeholder Communication: Acting as a bridge between stakeholders, communicating the system’s architectural design and explaining technical aspects.
- Documentation: Maintaining crucial technical documents such as design specifications and architecture diagrams.
How Generative AI can assist Software Architects?
Now let’s explore how Generative AI can support architects in their responsibilities.
Tech Stack Selection:
Generative AI can play a constructive role in this phase by recommending potential technologies and viable alternatives based on defined requirements.
For instance, consider the following prompt used to enumerate the comprehensive tech stack — including frontend, backend, infrastructure, security tools and CI/CD pipelines.
Rather than employing ChatGPT directly, we leveraged OpenAI’s chat API through Python for more efficient prompting. To learn more about this approach, check out the ChatGPT Prompt Engineering for Developers course.
import openai
import os
from dotenv import load_dotenv, find_dotenv
_ = load_dotenv(find_dotenv())
openai.api_key = os.getenv('OPENAI_API_KEY')
def get_completion(prompt, model="gpt-3.5-turbo"):
messages = [{"role": "user", "content": prompt}]
response = openai.ChatCompletion.create(
model=model,
messages=messages,
temperature=0
)
return response.choices[0].message["content"]
tech_stack_prompt = """
Your role is to act as a Software Architect assistant.
Requirements that needs to be met:
- Ecosystem: Node.js
- Cloud Provider: AWS
- Use a typed language
- Backend should be a RESTful API
- Use managed services provided by the cloud provider
- Use managed relational database provided by the cloud provider
- Prefer open source tools
- Do not suggest serverless functions such as AWS Lambda
- Prefer npm over yarn
Please suggest the below:
- Frontend Tech stack
- Programming language
- Framework
- Build tool
- Code coverage tool
- Dependency management
- Dependency vulnerability scanning
- Static code analysis
- Unit testing
- UI testing
- Backend API Tech stack
- Programming language
- Framework
- Build tool
- Code coverage tool
- Static code analysis
- Dependency management
- Dependency vulnerability scanning
- Unit testing
- API testing framework
- API documentation
- Database
- Secret management
- Security
- Container scanning tool
- Static Application Security Testing (SAST) tool
- Dynamic Application Security Testing (DAST) tool
- Penetration testing tool
- Infrastructure
- Infrastructure as code
- Security compliance scanning
- Logging
- Monitoring
- CI/CD tool
For each of the above, please provide in the below format:
<tool name>
Description: <short description>
Reason to choose: <reason> Pls note <reason> must not include generic words like popular or widely used or large community
Alternatives: <alternatives>. <provide the reasons to not choose them. Must not contain the reason that it lack some of the advanced features>.
Output must be in markdown syntax with proper newlines and indentation that can be directly copied to a markdown file.
"""
response = get_completion(tech_stack_prompt)
print(response)
GPT provided detailed recommendations for the tech stack (partial output shown below). The advantage of this approach is the reusability of these prompts across an organization, which expedites the architect’s work. As shown above, the tech stack is tailored to a Node.js environment hosted on AWS. However, this prompt can be customised according to the desired ecosystem and cloud service provider.
## Frontend Tech stack
### Programming language
TypeScript
**Description:** TypeScript is a typed superset of JavaScript that compiles to plain JavaScript.
**Reason to choose:** TypeScript provides type checking, which helps catch errors at compile time rather than runtime. This leads to more stable and maintainable code.
**Alternatives:** JavaScript. JavaScript lacks type checking, which can lead to errors at runtime.
### Framework
React
**Description:** React is a popular JavaScript library for building user interfaces.
**Reason to choose:** React has a large and active community, which means there are many resources and plugins available. It also has a virtual DOM, which makes it fast and efficient.
**Alternatives:** Vue.js, Angular. Vue.js is a newer framework with a smaller community. Angular is a more complex framework that can be difficult to learn.
### Build tool
Webpack
**Description:** Webpack is a module bundler for JavaScript applications.
**Reason to choose:** Webpack is highly configurable and can handle a wide range of tasks, such as bundling, minification, and code splitting. It also has a large and active community.
**Alternatives:** Parcel, Rollup. Parcel is a simpler build tool that is easier to set up, but it lacks some of the advanced features of Webpack. Rollup is a more specialized build tool that is designed for libraries rather than applications.
### Code coverage tool
Istanbul
**Description:** Istanbul is a code coverage tool for JavaScript applications.
**Reason to choose:** Istanbul is widely used and has good integration with other tools, such as Jest. It also has a large and active community.
**Alternatives:** nyc. nyc is a similar tool to Istanbul, but it is less widely used.
...
...
Technical Decision-Making:
Generative AI can support architects by presenting an array of options. For instance, during our experiment, we used this prompt to identify various deployment strategies for a React.js frontend on AWS.
deployment_options_prompt = """
What are the different ways to deploy the frontend react js application to AWS
- Suggest at least 3 ways
- For each way, provide the pros and cons
Suggest the best way among the 3 for the below requirements
- For a small team of 3 developers
- For faster deployment using AWS CloudFormation
- Lower cost
- Used by up to 1000 users
- Around 100 concurrent users
Output should be in markdown syntax with proper newlines and indentation that can be directly copied to a markdown file.
"""
response = get_completion(deployment_options_prompt)
print(response)
GPT provided these options with corresponding pros and cons and the recommended approach.
## Deploying React JS Application to AWS
There are several ways to deploy a React JS application to AWS. Here are three ways with their pros and cons:
### 1. Deploying to Amazon S3
#### Pros:
- Easy to set up and deploy
- Low cost
- Good for static websites
#### Cons:
- Limited functionality
- Not suitable for dynamic websites
### 2. Deploying to AWS Elastic Beanstalk
#### Pros:
- Easy to deploy and manage
- Supports multiple languages and frameworks
- Auto-scaling and load balancing
#### Cons:
- Higher cost than S3
- Limited control over infrastructure
### 3. Deploying to AWS EC2
#### Pros:
- Full control over infrastructure
- Can handle complex applications
- Can be cost-effective for larger applications
#### Cons:
- More complex to set up and manage
- Requires more technical expertise
- Higher cost than S3 and Elastic Beanstalk
### Best Way for the Given Requirements
Based on the requirements, the best way to deploy the React JS application to AWS would be to use AWS Elastic Beanstalk. It is easy to deploy and manage, supports auto-scaling and load balancing, and is cost-effective for a small team of 3 developers. It can handle up to 1000 users and around 100 concurrent users. Additionally, it can be accessed from anywhere in the world, making it suitable for a team working from different locations. Finally, AWS CloudFormation can be used to automate the deployment process, making it faster and more efficient.
System Design:
Large language models like GPT are trained on a myriad of architectural practices. As a result, they can suggest appropriate architectural designs tailored to a given project’s requirements. This not only significantly reduces the time spent on tech solution design but also provides architects with a foundational blueprint to kickstart their design.
For instance, Generative AI can expedite the process of creating deployment diagrams with the right prompts. In our experiment, we used the below prompt to generate the deployment diagram using DiagramGPT. Although the resulting design may not be the finest or most accurate, it offers a swift starting point.Please provide deployment diagram with all the below items
– React application deployed to s3 and exposed via cloudfront
– Backend nodejs service deployed using Elastic Beanstalk and communicate with AWS relation database
– Backend REST API exposed via API Gateway
– Cloudfront routing to s3 or api gateway based on path
– Route 53 in front of cloudfront
– Show the users accessing the route 53 from internet
– All Components should be inside the AWS Cloud
– Arrows to represent the network flow between the components
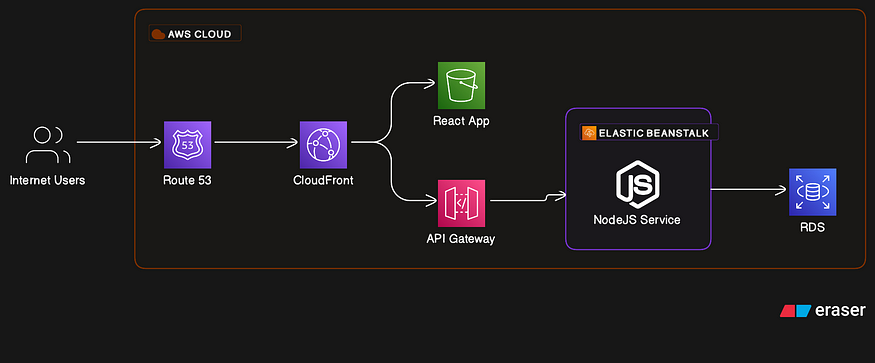
Cross-Functional Requirements:
Generative AI can assist architects in addressing cross-functional requirements by providing insights and recommendations. It’s worth noting that cross-functional requirements are often overlooked, particularly by less experienced or junior architects who are new to system design.
In such cases, using prompts as shown below as guidelines can help them navigate this critical aspect and ensure that these requirements are adequately considered in the architectural design process.
cross_functional_prompt = """
List all the cross-functional requirements (At least 15) that need to be followed in the overall system architecture
Information about the System architecture:
- Nodejs backend deployed in AWS Elastic Beanstalk
- React frontend deployed in AWS S3
- AWS RDS MySQL database
Each cross-functional requirement should be in the below format:
<requirement name>:
Description: <Description of the requirement in detail>
Best Practices: List of practices to follow to meet the requirement
Example: List multiple examples of how the requirement is met
Tools: List the tools that can be used to meet the requirement
The output must be in markdown syntax with proper new lines and indentation that can be directly copied to a markdown file
"""
response = get_completion(cross_functional_prompt)
print(response)
GPT was able to suggest detailed recommendations on various cross-functional requirements with tools and examples. Partial output below:
## Cross-Functional Requirements
-----------------
### Scalability:
Description: The system should be able to handle an increase in load without affecting performance.
Best Practices:
- Use horizontal scaling by adding more instances of the application.
- Use load balancers to distribute traffic evenly.
- Use caching to reduce the load on the database.
Example:
- Use AWS Elastic Beanstalk to automatically scale the application based on traffic.
- Use AWS Auto Scaling to add or remove instances based on CPU utilization.
Tools:
- AWS Elastic Beanstalk
- AWS Auto Scaling
-----------------
### Security:
Description: The system should be secure and protect against unauthorized access.
Best Practices:
- Use HTTPS for all communication.
- Use encryption for sensitive data.
- Use strong passwords and two-factor authentication.
Example:
- Use AWS Certificate Manager to obtain SSL/TLS certificates for HTTPS.
- Use AWS Key Management Service to encrypt and decrypt sensitive data.
Tools:
- AWS Certificate Manager
- AWS Key Management Service
-----------------
### Performance:
Description: The system should be performant and respond quickly to user requests.
Best Practices:
- Use caching to reduce the load on the database.
- Use CDNs to serve static assets.
- Use compression to reduce the size of responses.
Example:
- Use AWS ElastiCache to cache frequently accessed data.
- Use AWS CloudFront to serve static assets.
Tools:
- AWS ElastiCache
- AWS CloudFront
-----------------
### Monitoring:
...
### Availability:
...
### Reliability:
...
### Maintainability:
...
### Cost:
...
### Compliance:
...
### Disaster Recovery:
...
### Collaboration:
...
### Documentation:
...
### Performance Optimization:
...
### Accessibility:
...
Can Generative AI act as a full-fledged Architect?
If Generative AI is used as a full-fledged software architect, numerous consequential considerations arise.
Limitations in Design Detail:
Generative AI, in its current state, can only provide basic, high-level design blueprints but requires extensive detailing for more nuanced architectural decisions.
For instance, in our experimental project, when presented with the business requirements and tasks to design the system, GPT could only generate a very basic outline as shown below. This emphasises the need for careful prompting for each technical design element to achieve a comprehensive and customised architectural solution.
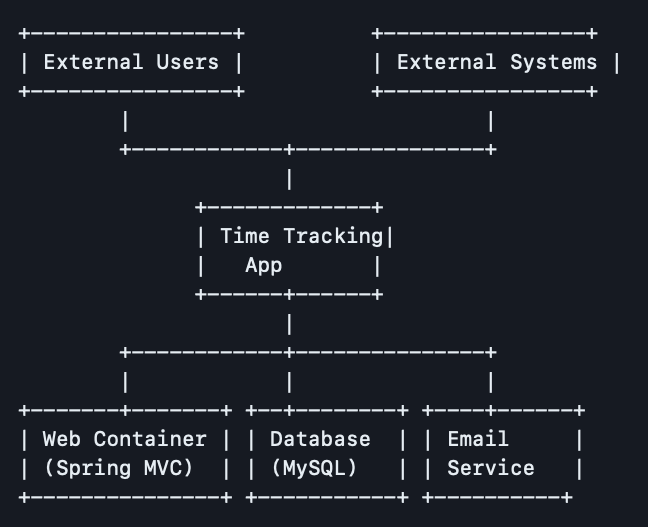
Complexity Overload:
When given more details, the AI started adding more complexity to the solution. This over-complication can make the development process harder than it needs to be. For example, it suggested a complex distributed architecture with serverless functions, which could be handled more effectively with a straightforward monolithic design.
Potential Security Risks:
The use of Generative AI in software architecture can pose certain security challenges. The AI might miss crucial aspects like securing the API endpoints, enforcing data encryption protocols, or overlooking vital network security measures such as firewalls or intrusion detection systems. These examples highlight the potential security blind spots in relying solely on generative AI for software architecture.
Constrained Creativity:
The innovative potential of AI is limited by the extent and variety of data it has been trained on. If the AI is tasked with architecting a software solution for cutting-edge technology, it may find it challenging to offer innovative solutions. The reason is, it might not have been trained on sufficient data pertinent to this field.
Overlooking Team and Culture Factors:
Generative AI might overlook team dynamics and organizational culture in its architectural suggestions. For example, it might propose a complex solution without considering the team’s abilities, or the availability of developers with needed skills. It could also suggest a solution that technically works but doesn’t align with the organization’s broader objectives.
A Tool for Architects, Not a Replacement
Ultimately, while Generative AI can be an invaluable tool, it’s not ready to replace human architects. The machine’s ability to make decisions is only as good as the data it’s been trained on. It cannot understand complex business contexts, foresee unanticipated challenges and bring in the creativity and innovation that a human architect can offer.
At the end of the day, machines cannot fully replace the decision-making capability of an experienced architect. Decisions in software architecture often involve subjective judgment, business intuition, and personal accountability — attributes that AI currently lacks.
So, for the foreseeable future, while AI will become an increasingly important tool in the architect’s toolbox, keep your architects close. Let them guide AI to best serve your software architecture needs. It’s through this collaboration that we’ll see the most effective and innovative solutions.